Coding guidelines?
General coding guidelines provide the programmer with a set of the best methods which can be used to make programs more comfortable to read and maintain. Most of the examples use the C language syntax, but the guidelines can be tested to all languages.
The following are some representative coding guidelines recommended by many software development organizations.
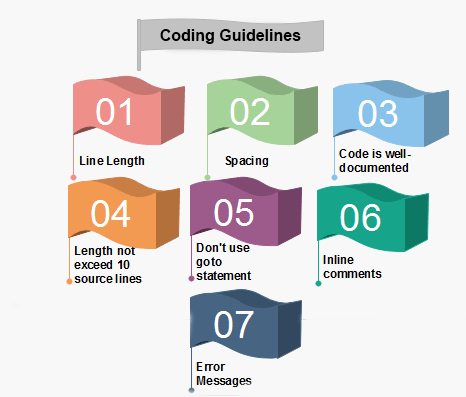
1. Line Length: It is considered a good practice to keep the length of source code lines at or below 80 characters. Lines longer than this may not be visible properly on some terminals and tools. Some printers will truncate lines longer than 80 columns.
2. Spacing: The appropriate use of spaces within a line of code can improve readability.
Example:
Bad: cost=price+(price*sales_tax)
fprintf(stdout ,"The total cost is %5.2f\n",cost);
Better: cost = price + ( price * sales_tax )
fprintf (stdout,"The total cost is %5.2f\n",cost);
3. The code should be well-documented: As a rule of thumb, there must be at least one comment line on the average for every three-source line.
4. The length of any function should not exceed 10 source lines: A very lengthy function is generally very difficult to understand as it possibly carries out many various functions. For the same reason, lengthy functions are possible to have a disproportionately larger number of bugs.
5. Do not use goto statements: Use of goto statements makes a program unstructured and very tough to understand.
6. Inline Comments: Inline comments promote readability.
7. Error Messages: Error handling is an essential aspect of computer programming. This does not only include adding the necessary logic to test for and handle errors but also involves making error messages meaningful.
Comments
Post a Comment